Blockly Blocks
Each block performs a different function, such as displaying a pop-up message or navigating to a new page in a SOTI Snap app.
You can connect blocks to other blocks to create more complicated logic statements. The shape of a block indicates where you can connect it to other blocks.
All available blocks are in the toolbox on the left side of the workspace. Click a block category to open a menu of blocks within that category. Hover your mouse pointer over a block or toolbox category to reveal a tooltip with a brief description of the block's use.To delete a block from the canvas, drag and drop it over the left toolbar. The toolbar turns red, and a trash icon appears.
Widget Values
- Get blocks pull in data from the specified widget.
- Set blocks dictate what should appear inside a widget, whether the value is fixed or dynamic (created by combining two or more values or blocks). You can also use a Set block to change the background Color of some widgets.
Open the Widget Values toolbox category to reveal a list of blocks related to all the widgets in your SOTI Snap app. Blocks are categorized by widget type.
You can set and get values for the following widgets:
- Button
- Checkbox
- Date
- Dropdown
- Layout
- Radio Button
- Rating
- Section
- Textbox
For instance, the following block can set the text of a Button widget. The widget's dropdown shows all the buttons in the app, organized by the pages where they appear. The button named button8 appears on the Submit Request page of the SOTI Snap app.
App Actions
Connections
Connection blocks contain hooks to inform when a request succeeds or fails so you can display the appropriate feedback on your app.
Click the Test button to map JSON key values to fields in a widget. In addition to assuring a proper connection to the data source, the test reveals the key values and adds them to the Response Data block. You can use these key values to map to fields in a widget. See the example below:
Code Blocks
Use Code Blocks to store custom JavaScript as a block for future use. Maintaining code blocks in your library will save time recreating complex or frequently used code. Drag and drop them on the canvas like other blocks.
XSight Topics
Global Variables
Read Global Variables for more information.
Local Variables
When creating a variable, Blockly adds new blocks to the Local Variables toolbox category to:
- Set the value of a variable
- Get the value of a variable
- Increment the value of a variable by 1 (default)
A complicated calculation that requires many Math blocks might be long and difficult to read. The example block below calculates the total price of a food order. All calculations to add the costs of each menu item are performed at once. The number of embedded calculations makes the block complex.
Logic
The If block runs its nested statement if the condition in its hexagonal block input is met. For instance, the coin toss script below prints a message saying "heads" if a randomly generated fraction is greater than 0.5.
TRUE
or FALSE
(Boolean) condition have a hexagonal shape.
Loops
i
Local Variable (see the
Local
Variables section) is incremented by one. The first
iteration prints the first element of the list called myList
. The
second iteration prints the second element of myList
, and so on.
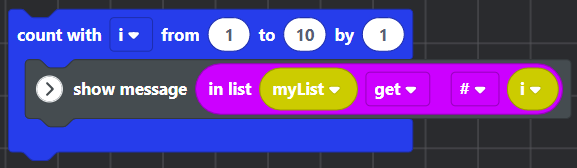
It is also common to loop through each item in a list. This is similar to how the block above loops through the first ten items.
The for each item in list block repeats its inner statement as many times as there are items in the list. At each new iteration of the loop, the specified variable has the value of the next item in the list.
The block below loops
through myList
and prints each list item.
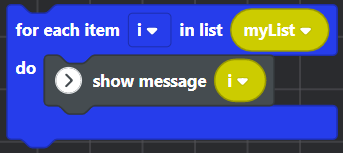
Math
Text
Lists
For instance, you can aggregate any number of names into a List block and then put the block within a Sort block to alphabetize them.
Color
#ff0064
(a pink color). Dates
Use Date blocks to work with SOTI Snap date widgets and to compare dates.
The example below displays an error if the date entered into a SOTI Snap Date widget is earlier than the current date.
Date blocks use the following format: month day
year
. For example, Jan 1 1970
.
Table
Use Table blocks to manage and access content within a Table widget. Capabilities include:
- Retrieving a value from a column in a table's selected row
- Adding a row to a table
- Renaming a table column
- Retrieving a column name
- Deleting a table row based on a column value
- Refreshing/updating the table view
- Running or cancelling record edits
- Connecting to an external data source to retrieve or add data
The following shows a Table block used to populate a table:
Debug Tools
The Log block returns the value of the block you wish to monitor as the app runs. Place the app in Preview mode and right-click anywhere on the page to see the information log. Open your web browser's Console view (The view's name and menu location vary by browser).
Debugging information is also available after the app is published. When testing a SOTI Snap app on a device, the Log block sends messages to the app's error log.
View error logs in the app by tapping
. SOTI Blockly runs on SOTI products only. Please contact the SOTI administrator.My Libraries
Use My Libraries to store a set of blocks for future use. Maintaining a block library will save time recreating complex or frequently used block structures. Drag and drop them on the canvas like other blocks, and modify them as required.